1. Giới thiệu về thêm một dữ liệu vào trong database
Select, insert, update, delete là những hành động mà chúng ta thường xuyên tương tác nhất trong quá trình quản trị hệ thống dữ liệu.
Hôm nay, chúng ta cùng nhau đề cập đến các vấn đề này nhé. Cập nhật dữ liệu một record vào trong database
Nguồn sử dụng
Nguồn sử dụng
- Database: northwind trong folder đính kèm
- Table: Employees
2. Thiết kế
Tạo trang mới đặt tên là updateRecord.aspx và thiết kế các control như sau
- Gridview control có id: gvData
- 5 Textbox control lần lượt có các id là: txtEmloyeeID, txtLastName, txtFirstName, txtTitle, txtCity
- 1 button có id: là btnUpdate & text là Cập nhật
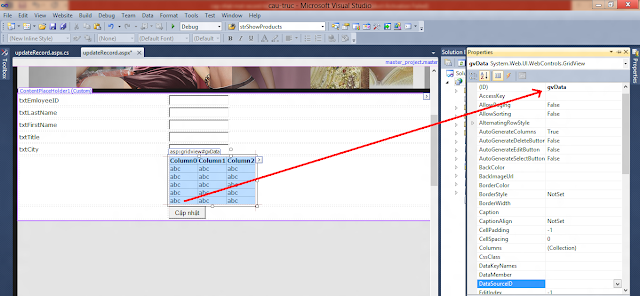
- Quan trọng: sử dụng đối tượng select trong gridview bằng cách chọn AutoGenerateSelectButton = true
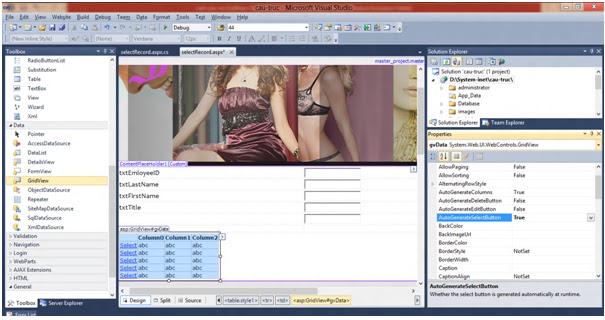
- Tạo sự kiện của button insert bằng cách double click vào nút button
1
2
3
| protected void btnUpdate_Click(object sender, EventArgs e) { } |
3. Coding
- Viết một phương thức loadEmployee() để load 10 records trong bảng Employees
1
2
3
4
5
6
7
8
9
10
11
| private void LoadEmployee() { string conn = "Data Source=.;Initial Catalog=Northwind;Integrated Security=True" ; SqlConnection sqlCon = new SqlConnection(conn); string GetEmployee = "Select top 10 * From Employees" ; SqlDataAdapter daCustomer = new SqlDataAdapter(GetEmployee, sqlCon); DataTable dtEmployee = new DataTable(); daCustomer.Fill(dtEmployee); gvData.DataSource = dtEmployee; gvData.DataBind(); } |
- Kết quả được hiển thị như sau:
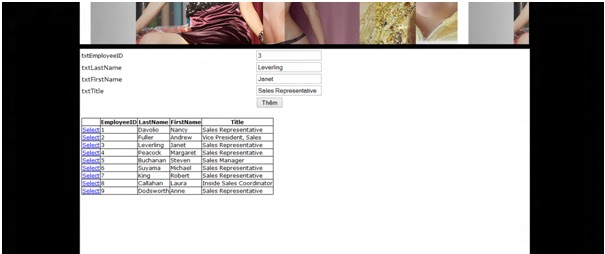
- Tạo một events (sự kiện) SelectedIndexChanging của đối tượng gridView và thực hiện coding như sau:
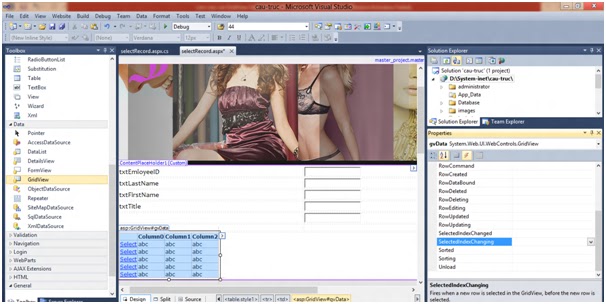
1
2
3
4
5
6
7
8
| protected void gvData_SelectedIndexChanging(object sender, GridViewSelectEventArgs e) { txtEmloyeeID.Text = gvData.Rows[e.NewSelectedIndex].Cells[1].Text; txtLastName.Text = gvData.Rows[e.NewSelectedIndex].Cells[2].Text; txtFirstName.Text = gvData.Rows[e.NewSelectedIndex].Cells[3].Text; txtTitle.Text = gvData.Rows[e.NewSelectedIndex].Cells[4].Text; txtCity.Text = gvData.Rows[e.NewSelectedIndex].Cells[5].Text; } |
- Viết một function update dữ liệu như sau
1
2
3
4
5
6
7
8
9
10
11
| void UpdateRecord() { string conn = "Data Source=.;Initial Catalog=Northwind;Integrated Security=True" ; SqlConnection sqlCon = new SqlConnection(conn); string UpdEmployee = "UPDATE Employees SET LastName = '" + txtLastName.Text + "', FirstName= '" + txtFirstName.Text + "', Title='" + txtTitle.Text + "' ,City='" +txtCity.Text+ "' WHERE EmployeeID=" + Convert.ToInt16(txtEmloyeeID.Text) + "" ; SqlDataAdapter daCustomer = new SqlDataAdapter(UpdEmployee, sqlCon); DataTable dtEmployee = new DataTable(); daCustomer.Fill(dtEmployee); gvData.DataSource = dtEmployee; gvData.DataBind(); } |
4. Giải thích
Function loadEmployee()
1
| string conn = "Data Source=.;Initial Catalog=Northwind;Integrated Security=True" |
Đoạn mã trên là chuỗi kết nối dữ liệu đến database là Northwind trong hệ quản trị dữ liệu SQL Server
Tạo đối tượng SQL Connection SqlCon
1
| SqlConnection sqlCon = new SqlConnection(conn); |
Đoạn mã truy vấn dữ liệu
1
| string GetEmployee = " Select EmployeeID,LastName,FirstName,Title From Employees" |
Đối tượng dùng xử lý dữ liệu
1
| SqlDataAdapter daCustomer = new SqlDataAdapter(GetEmployee, sqlCon); |
Tạo đối tượng DataTable chứa dữ liệu
1
| DataTable dtEmployee = new DataTable(); |
Fill dữ liệu vào DataTable
1
| daCustomer.Fill(dtEmployee); |
Và cuối cùng là hiển thị dữ liệu
1
2
| gvData.DataSource = dtEmployee; gvData.DataBind(); |
Sự kiện gvData_SelectedIndexChanging của gridview như sau
1
2
3
4
5
| txtEmloyeeID.Text = gvData.Rows[e.NewSelectedIndex].Cells[1].Text; txtLastName.Text = gvData.Rows[e.NewSelectedIndex].Cells[2].Text; txtFirstName.Text = gvData.Rows[e.NewSelectedIndex].Cells[3].Text; txtTitle.Text = gvData.Rows[e.NewSelectedIndex].Cells[4].Text; txtCity.Text = gvData.Rows[e.NewSelectedIndex].Cells[5].Text; |
- e.NewSelectedIndex: là sự kiện xảy ra tại dòng mà chúng ta đang chọn
- gvData.Rows[e.NewSelectedIndex] chính là sự kiện xảy ra tại bất kỳ record nào trên gridview mà bạn chọn nó
- gvData.Rows[e.NewSelectedIndex].Cells[4].Text là lấy giá trị tại ô thứ 4 tương ứng với column bạn chọn
Hàm UpdateRecord () dùng để thêm một record vào database với câu lệnh
1
| string UpdEmployee = "UPDATE Employees SET LastName = '" + txtLastName.Text + "', FirstName= '" + txtFirstName.Text + "', Title='" + txtTitle.Text + "' ,City='" +txtCity.Text+ "' WHERE EmployeeID=" + Convert.ToInt16(txtEmloyeeID.Text) + "" ; |
5. Kết quả
Kết quả đã được thêm vào database và sử dụng lại phương thức loadRecord để load mẫu tin vừa mới thêm vào
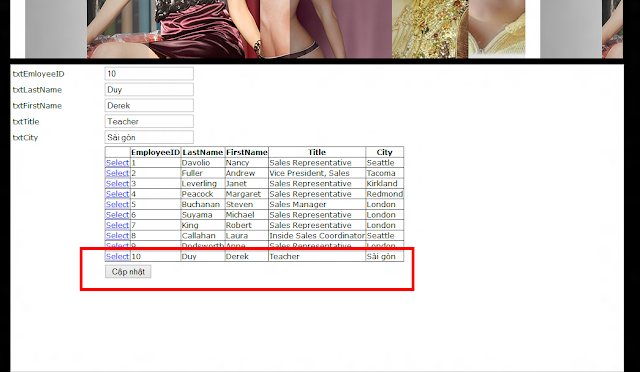
6. Source code đầy đủ của page
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
| using System; using System.Collections.Generic; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data.SqlClient; using System.Data; public partial class updateRecord : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { LoadEmployee(); } protected void btnUpdate_Click(object sender, EventArgs e) { UpdateRecord(); LoadEmployee(); } private void LoadEmployee() { string conn = "Data Source=.;Initial Catalog=Northwind;Integrated Security=True" ; SqlConnection sqlCon = new SqlConnection(conn); string GetEmployee = "Select top 10 EmployeeID,LastName,FirstName,Title,City From Employees" ; SqlDataAdapter daCustomer = new SqlDataAdapter(GetEmployee, sqlCon); DataTable dtEmployee = new DataTable(); daCustomer.Fill(dtEmployee); gvData.DataSource = dtEmployee; gvData.DataBind(); } protected void gvData_SelectedIndexChanging(object sender, GridViewSelectEventArgs e) { txtEmloyeeID.Text = gvData.Rows[e.NewSelectedIndex].Cells[1].Text; txtLastName.Text = gvData.Rows[e.NewSelectedIndex].Cells[2].Text; txtFirstName.Text = gvData.Rows[e.NewSelectedIndex].Cells[3].Text; txtTitle.Text = gvData.Rows[e.NewSelectedIndex].Cells[4].Text; txtCity.Text = gvData.Rows[e.NewSelectedIndex].Cells[5].Text; } void UpdateRecord() { string conn = "Data Source=.;Initial Catalog=Northwind;Integrated Security=True" ; SqlConnection sqlCon = new SqlConnection(conn); string UpdEmployee = "UPDATE Employees SET LastName = '" + txtLastName.Text + "', FirstName= '" + txtFirstName.Text + "', Title='" + txtTitle.Text + "' ,City='" +txtCity.Text+ "' WHERE EmployeeID=" + Convert.ToInt16(txtEmloyeeID.Text) + "" ; SqlDataAdapter daCustomer = new SqlDataAdapter(UpdEmployee, sqlCon); DataTable dtEmployee = new DataTable(); daCustomer.Fill(dtEmployee); gvData.DataSource = dtEmployee; gvData.DataBind(); } } |
Comments[ 0 ]
Đăng nhận xét